React/ReactJS: Using a jQuery Plugin with React
React already has a handful of useful UI components for most of our front-end needs; the official site maintains a list here. Also, there is Reactstrap which provides ready-to-use stateless Bootstrap 4 components like accordions, datepickers, modals, tooltips, etc., compatible wth React 16+.
But a developer sometimes fancy a plugin created for a much older library like jQuery and wants to use it in his/her React project (of course, time congestion very often necessitates such unwarranted move). This tutorial will show you how to use jQuery with React and on using jQuery plugins with React in general.
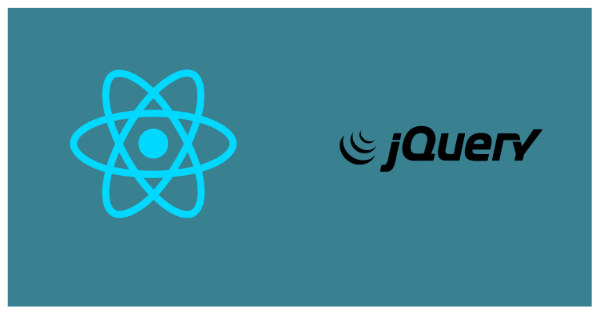
For our example, we pick a simple jQuery plugin called jCirclize, which loads a given percentage value in circle. We will learn how to import the plugin and use it inside a React component.
The first thing to do is to install the jQuery package using npm.
npm install jquery

Next go to jCirclize's Github repository and download the ZIP file. On completion of download, extract/unzip the ZIP file, copy the files jCirclize.css
and jCirclize.js
and place them inside proper directories in your project, say, /css
and /js
.
Import them (along with jQuery) into the file where your component would be.
import $ from 'jquery';
import './css/jCirclize.css';
import './js/jCirclize.js';
We create a new component called <Percentage/>
and arrange the code for using a jQuery plugin similar to what is documented in React's official site here. The plugin is destroyed inside the componentWillUnmount()
method, which happens whenever the user moves away from the component. There is a callback ref referencing the <div>
element.
class Percentage extends Component {
componentDidMount() {
this.$el = $(this.el);
this.$el.circlize();
}
componentWillUnmount() {
this.$el.circlize('destroy');
}
render() {
return (
<div ref={el => this.el = el}>
</div>
);
}
}
export default Percentage;
Now if you try to render this <Percentage/>
component, the console will throw an error 'jQuery is not defined no-undef
'.
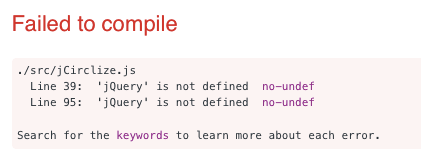
To fix this, open the jCirclize.js
file and paste the below import statement at the very beginning of the file.
import jQuery from 'jquery';
But in other jQuery plugins, the error could instead read '$ is not defined no-undef
'. In such cases, just replace 'jQuery
' with '$
' in the import statement.
import $ from 'jquery';
On successful rendering of the component, the plugin loads as below.
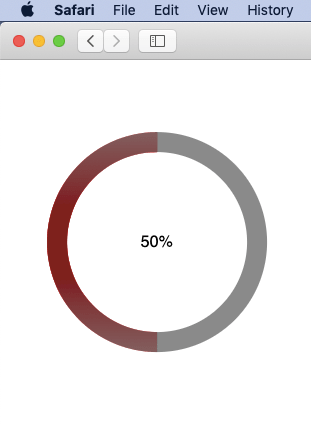
Options for the jCirclize Plugin
Now this section is about customizing the plugin and is not much related to the topic anymore. And should the plugin serve no purpose for your project, you can scroll up.
We will change the stroke (the thickness of the circle), the percentage value and the background colour of the circle. As in most customizable jQuery plugins, the options are passed as property-value pairs of an object literal to the plugin method.
class Percentage extends Component {
componentDidMount() {
this.$el = $(this.el);
this.$el.circlize({
stroke: 15,
percentage: 45,
usePercentage: true,
background: "#1abc9c",
gradientColors: ["#ffa500", "#ff4500", "#ffa500"]
});
}
componentWillUnmount() {
this.$el.circlize('destroy');
}
render() {
return (
<div ref={el => this.el = el}>
</div>
);
}
}
export default Percentage;
We get our customized circular percentage loader.
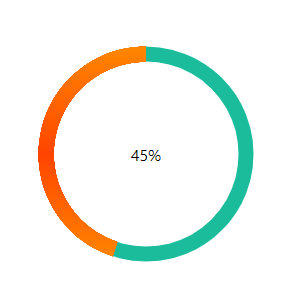