React/ReactJS: Add Class Conditionally
Often in web applications we come across situations like the need to hide an element till some condition is met or change the background colour of an option when it is selected. Sometimes, the need is to add an active class on click of a button. We come across many such requirements which all can be achieved by adding of some class conditionally.
In this tutorial, we will be learning how in React we can add a class name dynamically upon meeting some condition.
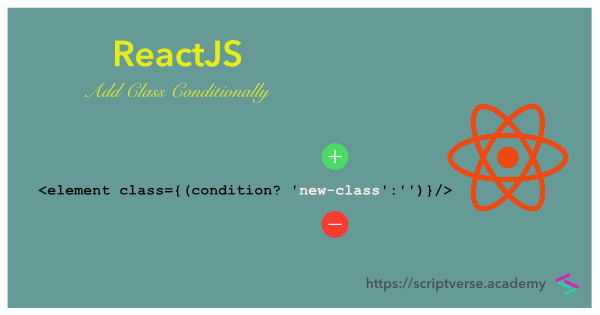
Consider the below <div/>
element which wraps the following loader image.
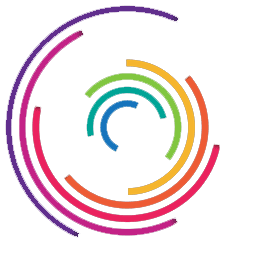
render() {
return (
<div className="loader">
<img src="https://i.redd.it/o6m7b0l6h6pz.gif"/>
</div>
);
}
Now say you want to hide the spinner upon successful fetching of some data from an API inside your componentDidMount()
. To achieve that, we can actually toggle the below .hide
class by adding/removing it to/from the wrapper <div/>
element.
.hide {
display: none
}
Now to toggle the class, you can maintain some state variable, say fetchSuccess
, which is initialized to a boolean value false
inside the constructor()
and set it to true
upon completion of request. We can then set a conditional statement inside a pair of braces as follows:
render() {
return (
<div className={"loader " + (this.state.fetchSuccess? "hide":"")}>
<img src="https://i.redd.it/o6m7b0l6h6pz.gif"/>
</div>
);
}
Carefully note the space after the loader
class.
The below section has a full working example component showing how in React we can conditionally add a classname.
Adding Class Conditionally
We will be using the browser's built-in Promise-based Fetch API to request external JSON data asynchronously from https://jsonplaceholder.typicode.com/users. As soon as the fetch has a response, the fetchSuccess
state variable is set to true
. The conditional statement is then checked and the .hide
class is added to the wrapper <div/>
and the spinner is hidden.
class LoaderExample extends React.Component {
constructor(props) {
super(props);
this.state = {
fetchSuccess: false
}
}
componentDidMount() {
fetch('https://jsonplaceholder.typicode.com/users')
.then(response => {
this.setState({
fetchSuccess: true
});
});
}
render() {
const{ fetchSuccess } = this.state;
return (
<div className={"loader " + (fetchSuccess? "hide":"")}>
<img src="https://i.redd.it/o6m7b0l6h6pz.gif"/>
</div>
);
}
}
export default LoaderExample;