React/ReactJS: HTTP Requests using Fetch API
and axios
In this tutorial, we will learn how to make simple HTTP requests or API calls in React with examples.
In the first section, we will be using the browser's in-built Fetch API to request external data asynchronously.
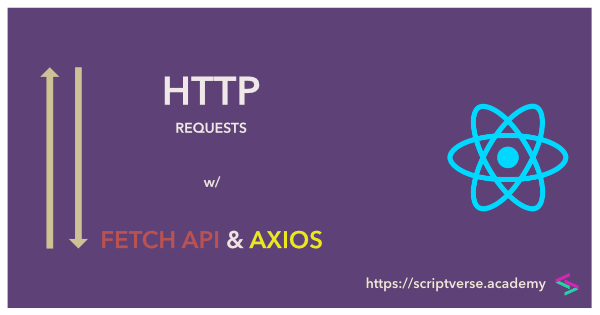
Fetch API is Promise-based and has the fetch()
method which is defined on the window
object. Fetch is supported in the latest versions of Chrome, Firefox, Safari and Opera. However, it is not supported in IE 11. To make it work there, you have to include some polyfills like isomorphic-fetch.
But a little reminder before we start. In React, AJAX calls should be made inside the componentDidMount()
lifecycle method and not inside the about-to-be-deprecated componentWillMount()
. The differences between the two have been covered here.
Fetching Data with Fetch API
And so we fetch the data inside componentDidMount()
from an external source, the free online REST API for fake data: https://jsonplaceholder.typicode.com/users. The code inside componentDidMount()
is plain JavaScript, except for the assigning setState()
method.
class Users extends React.Component{
constructor(props) {
super(props);
this.state = {
users: []
}
}
componentDidMount() {
fetch('https://jsonplaceholder.typicode.com/users')
.then(response => response.json())
.then(users => this.setState({ users }));
}
render() {
return (
<div>
<ul>
{
this.state.users.map(usr =>
<li key={usr.id}>{usr.name}</li>
)
}
</ul>
</div>
);
}
}
export default Users;
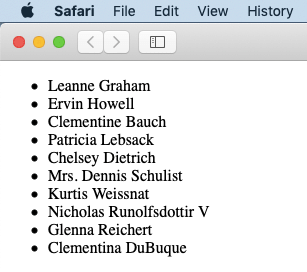
Fetching Data wth Axios
In this section, we explore another Promise-based HTTP client called axios
that works in both the browser and the Node.js environment. The advantage (compared to the fetch()
method above) is that, unlike the Fetch API, axios
works well in IE11. You can check for the supported browsers here.
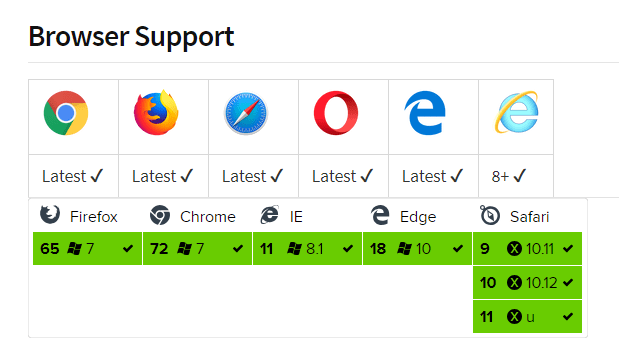
Install the package using npm
.
npm install axios --save
Import it into the component where you intend to make the HTTP request.
import axios from 'axios';
As recommended in React, we make the request inside componentDidMount()
.
class Users extends React.Component{
constructor(props) {
super(props);
this.state = {
users: []
}
}
componentDidMount() {
axios.get('https://jsonplaceholder.typicode.com/users')
.then(response => response.data)
.then(users => this.setState({ users }))
}
render() {
return (
<div>
<ul>
{
this.state.users.map(usr =>
<li key={usr.id}>{usr.name}</li>
)
}
</ul>
</div>
);
}
}
export default Users;

The data
(the JSON data we are looking for) comes as a property in the response object along with other properties like config
, headers
, request
, status
and statusText
. That is why, unlike in the fetch()
method above, we use response.data
while making the assignment in setState()
.
It is always a good practice to attach the catch()
handler.
componentDidMount() {
axios.get('https://jsonplaceholder.typicode.com/users')
.then(response => response.data)
.then(users => this.setState({ users }))
.catch(err => console.log(err))
}