PHP Sort Arrays
In this tutorial, we will be exploring the various sort functions available in PHP for arrays. These functions give us the provision to sort an array by values or by keys in both ascending and descending order.
First, we will look at the functions which sort a given array into ascending order.
Ascending Order, Low to High
The first is the sort()
function.
sort()
The sort()
function sorts a given array in ascending order. When this function is applied on an array, the elements are reordered from lowest to highest.
Consider an array consisting of the first four version names of the elementaryOS operating system (based on Ubuntu).
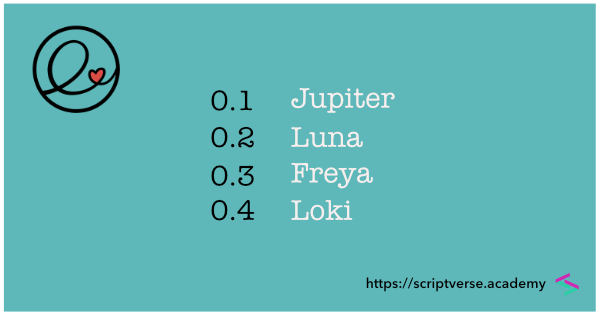
<?php
$eos = array('Jupiter', 'Luna', 'Freya', 'Loki');
?>
We apply the sort()
function on it, and print the resulting array.
<?php
$eos = array('Jupiter', 'Luna', 'Freya', 'Loki');
sort($eos);
print_r($eos);
?>
The resulting sorted array gets printed as
<?php
Array ( [0] => Freya [1] => Jupiter [2] => Loki [3] => Luna )
?>
NOTE: The sort()
function sorts a given array in ascending alphanumeirc order [a-z 0-9]
. If your array has both text and numeric values, the numeric values will come last after sorting.
We recall that all arrays are stored as associative arrays internally in PHP. The sort()
function does not consider the keys; they are discarded. To understand this, we modify the above array by assigning customs key values (which are versions) to the values.
<?php
$eos = array(
'0.1' => 'Jupiter',
'0.2' => 'Luna',
'0.3' => 'Freya',
'0.4' => 'Loki'
);
sort($eos);
print_r($eos);
?>
We have assigned our custom keys, but those keys are discarded and the following array gets printed.
<?php
Array ( [0] => Freya [1] => Jupiter [2] => Loki [3] => Luna )
?>
asort()
Now if we need to retain the original assigned keys, we need to use the asort()
function. This function sorts the given array in ascending order, all the while maintaining their original index association.
We make use of the above array as an example. The following script
<?php
$eos = array(
'0.1' => 'Jupiter',
'0.2' => 'Luna',
'0.3' => 'Freya',
'0.4' => 'Loki'
);
asort($eos);
print_r($eos);
?>
will print the following
<?php
Array ( [0.3] => Freya [0.1] => Jupiter [0.4] => Loki [0.2] => Luna )
?>
We notice that the original keys are not discarded as in the sort()
function; they remain associated with their respective values.
ksort()
Now there is another function called ksort()
which sorts a given array in ascending order by keys. As the array we have used in the above two examples is already sorted by keys, we will juggle it and get it sorted by values. We see that the keys are not ordered this time.
We apply the ksort()
function on it
<?php
$eos = array(
'0.3' => 'Freya',
'0.1' => 'Jupiter',
'0.4' => 'Loki',
'0.2' => 'Luna'
);
ksort($eos);
print_r($eos);
?>
and the array gets sorted by keys in ascending order
<?php
Array ( [0.1] => Jupiter [0.2] => Luna [0.3] => Freya [0.4] => Loki )
?>
natsort()
We consider a different array here. The elements are some PDF file names involving numbers.
<?php
$pdf = array('php1.pdf', 'php11.pdf', 'php2.pdf', 'php12.pdf');
?>
If you use the sort()
function to sort the above array, it will not sort it as you would intend to. You would have expected php2.pdf
to come before php11.pdf
, but no it doesn't; it gets sorted as follows.
<?php
Array ( [0] => php1.pdf [1] => php11.pdf [2] => php12.pdf [3] => php2.pdf )
?>
This is where the natsort()
function comes in handy; it sorts a given array using a natural order algorithm. If we use natsort()
to sort the above array
<?php
$pdf = array('php1.pdf', 'php11.pdf', 'php2.pdf', 'php12.pdf');
natsort($pdf);
print_r($pdf);
?>
we get the desired sorted result as
<?php
Array ( [0] => php1.pdf [2] => php2.pdf [1] => php11.pdf [3] => php12.pdf )
?>
natcasesort()
Now there is one important thing to note when it involves the case of letters: the uppercased letters come first in sorting order. We capitalize the first letters of the last two elements in the below array and use natsort()
to sort them.
<?php
$pdf = array('php1.pdf', 'php11.pdf', 'Php2.pdf', 'Php12.pdf');
natsort($pdf);
print_r($pdf);
?>
In the resulting sort, the capitalized elements occur first, irrespective of the numbers within them. We do not get a properly sorted array.
<?php
Array ( [2] => Php2.pdf [3] => Php12.pdf [0] => php1.pdf [1] => php11.pdf )
?>
To get a properly sorted arrray, we use the natcasesort()
function here, which uses a case insensitive natural sorting algorithm.
<?php
$pdf = array('php1.pdf', 'php11.pdf', 'Php2.pdf', 'Php12.pdf');
natcasesort($pdf);
print_r($pdf);
?>
This time, the array is sorted properly.
<?php
Array ( [0] => php1.pdf [2] => Php2.pdf [1] => php11.pdf [3] => Php12.pdf )
?>
Descending Order, High to Low
In this section, we will explore the functions which does the sorting from the highest to the lowest, i.e., in descending order.
rsort()
The rsort()
function sorts a given array in descending order. When this function is applied on an array, the elements are reordered from the highest to the lowest.
We take the same array we use for the sort()
function and apply rsort()
on it.
<?php
$eos = array('Jupiter', 'Luna', 'Freya', 'Loki');
rsort($eos);
print_r($eos);
?>
The above script prints the below sorted array
<?php
Array ( [0] => Luna [1] => Loki [2] => Jupiter [3] => Freya )
?>
Similar to sort()
, the rsort()
function also does not consider the assigned keys; they are discarded. Below we assign our own custom keys (which are eOS versions) to the values
<?php
$eos = array(
'0.1' => 'Jupiter',
'0.2' => 'Luna',
'0.3' => 'Freya',
'0.4' => 'Loki'
);
rsort($eos);
print_r($eos);
?>
But upon sorting, the values get reassigned to the default indices, starting from 0
<?php
Array ( [0] => Luna [1] => Loki [2] => Jupiter [3] => Freya )
?>
arsort()
Now unlike rsort()
, the arsort()
function keeps the original keys assigned to the respective values; they remain unchanged after sorting.
We make use of the above same array as an example. The following script
<?php
$eos = array(
'0.1' => 'Jupiter',
'0.2' => 'Luna',
'0.3' => 'Freya',
'0.4' => 'Loki'
);
arsort($eos);
print_r($eos);
?>
will print the following
<?php
Array ( [0.2] => Luna [0.4] => Loki [0.1] => Jupiter [0.3] => Freya )
?>
Note that the original keys remain the same unlike in the case of the rsort()
function.
krsort()
Now the krsort()
function sorts a given array by keys in descending order. We juggle the above array and take it as already sorted in ascending order. Note the unordered keys.
Upon applying the krsort()
function on it
<?php
$eos = array(
'0.3' => 'Freya',
'0.1' => 'Jupiter',
'0.4' => 'Loki',
'0.2' => 'Luna'
);
krsort($eos);
print_r($eos);
?>
the array gets sorted by keys in descending order as follows
<?php
Array ( [0.4] => Loki [0.3] => Freya [0.2] => Luna [0.1] => Jupiter )
?>
Notes
-
The
sort()
,asort()
andksort()
functions sort arrays in ascending alphanumeric order [a-z 0-9]. If an array containing both string and number values are sorted, the numbers come last. Similarly, thersort()
,arsort()
andkrsort()
functions sort arrays in descending alphanumeric order [9-1 z-a].