PHP Arrays
An array is a collection of related data items. In PHP, an array is a collection of key => value
pairs, separated by commas.
The array()
Construct

Consider three colours Red (), Yellow (), and Blue (). Let us construct a key => value
mapping with integer keys (starting with 0) mapped to their names as values. This mapping can be represented as an array in PHP using the array()
construct assigning it to a variable $colours
as shown below
<?php
$colours = array(
0 => 'Red',
1 => 'Yellow',
2 => 'Blue');
?>
An array key should either be an integer or a string, but the corresponding mapped value can be any PHP type, including another array.
String keys give us the provision to describe an array better. Choose a particular colour from the above array, say, Red (). We can create another array to describe its other associated properties picking meaningful key names like name
, hex
, and rgb
<?php
$colour = array(
'name' => 'Red',
'hex' => '#ff0000',
'rgb' => 'rgb(255,0,0)');
?>
Generally, keys are optional in PHP. But when keys are explicitly specified as in the above two example arrays, they are known as asociative arrays. And if the keys/indices of an array are sequential integers starting with 0, we need not specify the keys explicitly; we can just write as
<?php
$colours = array('Red', 'Yellow', 'Blue');
?>
Such arrays are generally called indexed or numeric arrays, though internally PHP stores all arrays as associative arrays, the difference being only in what the keys happen to be.
As of PHP 5.4, we can do away with the array()
and use just []
<?php
$colours = ['Red', 'Yellow', 'Blue'];
?>
Accessing Array Elements
We may access an array value (or element) for either reading, assignment or modification using the array's variable name followed by square backets with the element's key or index within.
Reading Array Elements
For example, the first element of the array $colours
with index 0 can be accessed as
<?php
echo $colours[0]; // Red
?>
and the hex code of Red can be accessed from the array $colour
using the key hex
as
<?php
echo $colour['hex']; // #ff0000
?>
The print_r()
Function
We can use the print_r()
function to print out the whole array in a readable manner. The following statement
<?php
print_r($colour);
?>
will print out the array $colour
in the following way
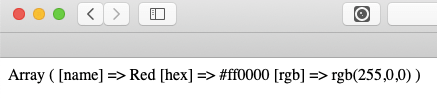
Assignment
We need not store all key => value
pairs of an array upon declaration. As with variables, we can also assign array members directly with =
by referencing its keys. The above two example arrays can also be constructed as shown below
<?php
$colours[0] = 'Red';
$colours[1] = 'Yellow',
$colours[2] = 'Blue';
?>
<?php
$colour['name'] = 'Red';
$colour['hex'] = '#ff0000',
$colour['rgb'] = 'rgb(255,0,0)';
?>
Modifying Array Elements
Now say we want to overwrite the details of Red () with the details of another colour Yellow (). We can just do so by reassigning the values as
<?php
$colour['name'] = 'Yellow';
$colour['hex'] = '#ffff00',
$colour['rgb'] = 'rgb(255,255,0)';
?>
Traversing an Array with foreach
PHP has the foreach
loop construct to iterate over arrays. This construct works only on arrays and objects.
Here is the short example of using foreach
to traverse our first array $colours
<?php
foreach($colours as $key => $value) {
echo $key.") ".$value."<br/>";
}
?>
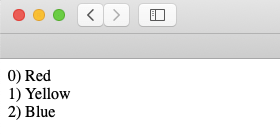
where $key
and $value
have their usual meanings and $key
is optional.
Assigning in a Single Operation with list()
The list()
construct allows us to assign array values to variables in a single operation. list()
works only on numeric arrays. In the following example, we assign the elements of array $colours
to variables $r
, $y
and $b
<?php
list($r, $y, $b) = $colours;
echo "$r, $y and $b are few examples of colours.";
?>
Multidimensional Arrays
There can be arrays within arrays, which are known as nested or multidimensional arrays. We can store details of all three colours we have covered so far - Red, Yellow and Blue - in the following way
<?php
$colour = array(
array(
'name' => 'Red',
'hex' => '#ff0000',
'rgb' => 'rgb(255,0,0)'
),
array(
'name' => 'Yellow',
'hex' => '#ffff00',
'rgb' => 'rgb(255,255,0)'
),
array(
'name' => 'Blue',
'hex' => '#0000ff',
'rgb' => 'rgb(0,0,255)'
)
);
?>
Notes
-
array()
is an empty array.