PHP: Superglobals
Generally, PHP does not support global variables in a way other languages do.
However, there exists certain predefined variables within PHP which behave like them. They are automatically accessible from any scope. These variables are known as superglobals and are listed below.
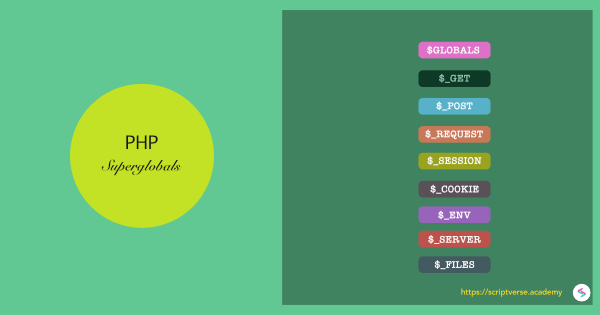
We go through each of them briefly in the sections below.
$GLOBALS
Unlike in other programming languages, there is no support for global variables in PHP. Variables are local to their scope. For example, a variable defined outside a function is not accessible inside the function and the vice versa.
Consider the below script, where the variable $pi
is declared outside the function. We try to return $pi
inside the get_pi()
function. It does not happen. The script does not output anything.
<?php
$pi = 3.141;
function get_pi() {
return $pi;
}
echo get_pi();
?>
There is this earlier workaround to fix this using the global
keyword and its unrecommended practice to declare the variable as global and import it into the function's scope. The below script will print 3.141.
<?php
$pi = 3.141;
function get_pi() {
global $pi;
return $pi;
}
echo get_pi();
?>
However, using the global keyword is discouraged. The correct way would be to use $GLOBALS, an associative array containing references of all variables defined in the global scope, as shown in the script below. The variable names are the keys of the $GLOBALS array.
<?php
$pi = 3.141;
function get_pi() {
return $GLOBALS['pi'];
}
echo get_pi();
?>
The above script outputs 3.141.
$_GET
The $_GET
superglobal is an associative array of all the GET
variables that the current PHP script receives from the browser via the URL parameters. Usually, they are sent from a form with method="get"
, and the values are exposed on the browser's address bar. Suppose the value of the name
variable is passed via the URL as
http://startrek.com/?name=Jim
You can make use of the $_GET
superglobal to access the passed variable and its value. The portion of the string after the URL ?name=Jim
is called query string.
<?php
echo 'Hello, ' . htmlspecialchars($_GET["name"]) . '!';
?>
The above script will output something like:
Hello, Jim!
$_POST
$_POST
is an associative array of all the POST
variables that the current PHP script receives from the client browser. Unlike in GET
method, the variables are not passed through the URL.
If in the form of the previous page the <input name="name"/>
element's filled value was Jim
, then the value can be accessed via the global $_POST
array in the current script as follows:
<?php
echo 'Hello, ' . htmlspecialchars($_POST["name"]) . '!';
?>
The script will output something like:
Hello, Jim!
$_SERVER
$_SERVER
is one useful superglobal containing information on headers, paths, server addresses, script locations and such. It is an associative array with its key-value pairs created by the web server. It contains many useful elements like SERVER_ADDR
, DOCUMENT_ROOT
, PHP_SELF
, HTTP_USER_AGENT
, 'REMOTE_ADDR
, etc. You can print out the entire key-value pairs in $_SERVER
using print_r()
<?php
print_r($_SERVER);
?>
As an example, here we get the HTTP_USER_AGENT
element, which fetches the details of your web browser.
<?php
echo $_SERVER['HTTP_USER_AGENT'];
?>
If you use Safari, the above script will output something like:
Mozilla/5.0 (Macintosh; Intel Mac OS X 10_14_4) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/12.1 Safari/605.1.15
$_SESSION
The $_SESSION
superglobal is an associative array of session variables which are available in the current script.
A session is usually set after the session_start()
function.
<?php
session_start();
$_SESSION['city'] = 'Shillong';
?>
If a session is set, it can be accessed throughout the script via the $_SESSION
superglobal
<?php
if (isset($_SESSION['city']))
echo htmlspecialchars($_SESSION['city']);
?>
$_COOKIE
The $_COOKIE
superglobal is an associative array of cookie variables, set by the setcookie()
function, which are passed to the current script.
If a cookie called browser
is set with the expiry time to 1 day, as follows,
<?php
$browser = "Safari";
setcookie("browser", $browser, time()+60*60*24);
?>
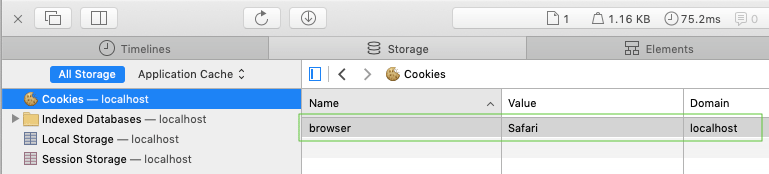
it can be accessed in the next target page with the $_COOKIE
superglobal.
<?php
if (isset($_COOKIE['browser']))
echo htmlspecialchars($_COOKIE['browser']);
?>
$_REQUEST
The $_REQUEST
superglobal contains the contents of $_GET
, $_POST
and $_COOKIE
built-in arrays, merged into one. So the form data sent by method="get"
or method="post"
to the posted page can also be retrieved by $_REQUEST
.
Here is a simple form with one input field.
<form name="newsletter" method="post" action="newsletter.php">
<input type="email" name="email"/>
<input type="submit" value="Submit"/>
</form>
On submission, in the target newsletter.php
page, the posted data (which is the value of the form element <input/>
, with its name as email
) can be retrieved by $_REQUEST
with the key as the name
attribute
<?php
echo $_REQUEST['email'];
?>
$_ENV
The $_ENV
superglobal contains an array of information about your environment.
The phpinfo()
function comes in handy to check the available environment variables
<?php
echo phpinfo();
?>
which are tabulated under the Environment section.
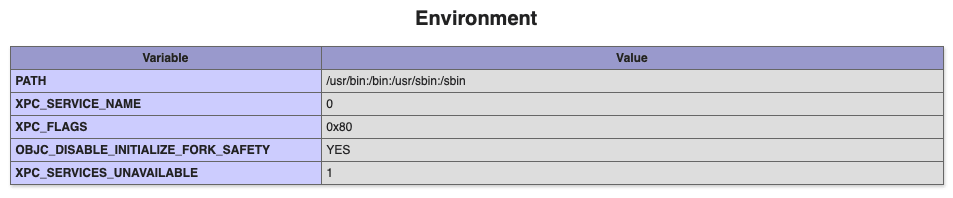
Or you can just print out the entire array as
<?php
print_r($_ENV);
?>
Array ( [PATH] => /usr/bin:/bin:/usr/sbin:/sbin
[XPC_SERVICE_NAME] => 0 [XPC_FLAGS] => 0x80
[OBJC_DISABLE_INITIALIZE_FORK_SAFETY] => YES
[XPC_SERVICES_UNAVAILABLE] => 1 )
To get the value of a certain environment variable, pass it as key to the $_ENV
array
<?php
echo $_ENV['PATH'];
?>
You can also use the getenv()
function to get the value of an environment variable.
<?php
echo getenv['XPC_FLAGS'];
?>
$_FILES
PHP is able to receive any file uploaded in an RFC-1867 compliant browser. The $_FILES
superglobal contains the contents of the files uploaded to the current script via the POST method.
The form below has one file upload field, which posts into the current page on submit.
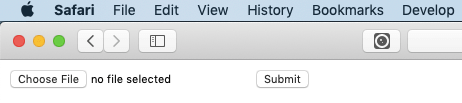
<form action="<?php echo $_SERVER['PHP_SELF']; ?>"
method="post" enctype="multipart/form-data">
<input type="file" name="file"/>
<input type="submit" value="Submit"/>
</form>
We add a little script above to print out the contents of the uploaded file/files.
<?php
if(sizeof($_FILES) > 0)
print_r($_FILES);
?>
As an example, here I upload a PDF file called Bucket Sort.pdf
(of course, you can upload other files like images). On pressing the Submit
button, the $_FILES
superglobal reveals the details of the uploaded file like name
, type
, size
, tmp_name
and error
.
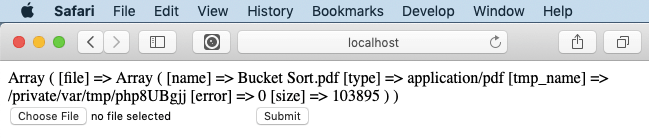
Notes
- Sensitive data should not be passed via the URL.
-
The superglobals are available in all scopes throughout the script. Declaring any of them with the
global
keyword is unnecessary to access functions/methods.