PHP: isset()
, unset()
and empty()
PHP has three language constructs to manage variables: isset()
, unset()
and empty()
. We take a look at each of them separately in the below sections.
PHP isset()
The isset()
language construct checks whether a certain variable has been previously set or not and returns a boolean value: true
if set, false
if not or set to null
.
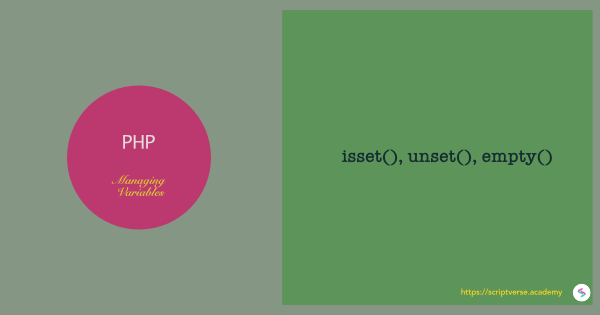
In the following script, we assign the variable $hello
to the string 'HELLO'
. Inside the if
statement, isset()
is applied on $hello
to check whether it is set or not
<?php
$hello = 'HELLO';
if(isset($hello)) {
echo $hello. ' is set.'; // HELLO is set.
}
?>
A variable set to an empty string is also set, and hence, isset()
returns true
$empty = '';
if(isset($empty)) {
echo $empty. ' is also set.'; // is also set.
}
A variable set to a false
value is also set, and hence, in this case too isset()
returns true
$untrue = false;
if(isset($untrue)) {
echo $untrue. ' is also set.'; // is also set.
}
Multiple variables can also be passed as arguments to isset()
, but it will return true
only if all the supplied arguments are set
$hello = 'HELLO';;
$empty = '';
$untrue = false;
if(isset($hello, $empty, $untrue)) {
echo $hello, $empty, $untrue, ' are all set.';
}
PHP unset()
The unset()
construct destroys a given variable/set of variables. Let us take the very first example used in the above section and apply unset()
on the variable $hello
just after it is assigned to 'HELLO'
. As we call isset()
on the variable $hello
, which was just unset()
, it returns false
<?php
$hello = 'HELLO';
unset($hello);
if(isset($hello)) { // the condition fails; echo is not executed
echo $hello. ' is set.';
}
?>
Several variables can be destroyed/unset at a time
<?php
$hello = 'HELLO';
$n = 1;
$bool = false;
unset($hello, $n, $bool);
?>
PHP empty()
The empty()
construct returns true
if a variable is set to an empty string, to 0 (either as a number or a string), to false
, or to null
<?php
$hello = '';
$zero = 0;
$raining = false;
$nihil = null;
if(empty($hello)) { // true
echo 'true';
}
if(empty($zero)) { // true
echo 'true';
}
if(empty($raining)) { // true
echo 'true';
}
if(empty($nihil)) { // true
echo 'true';
}
?>
However, a space character is not considered empty. In the following code, we set the variable $space
to a single space character. Applying empty()
on it, the if
condition fails
<?php
$space = ' '; // single space
if(empty($space)) { // the condition fails; echo is not executed
echo $space. ' is empty.';
}
?>