React/ReactJS: Add React/ReactJS to an HTML File
Your need not go about creating a full-fledged ReactJS application or even a boilerplate (as we did in our previous tutorial) to try out ReactJS; you can actually just add and use ReactJS in a normal HTML file without typing terminal commands and installing myriads of npm
packages — it is as simple as adding a jQuery file.
Also it is much more convenient for those inclined to using the browser's console to just type-in and see the outputs of the objects inside to the React.createElement()
and ReactDOM.render()
functions.
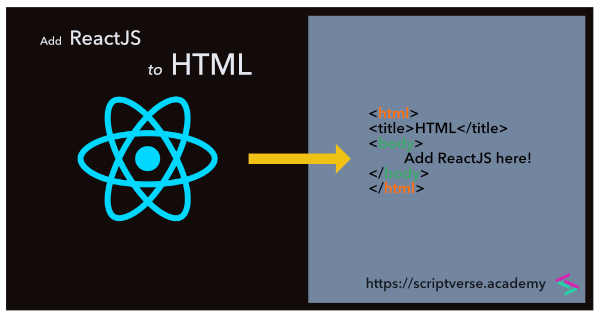
Add React Component to HTML
We need three <script>
tags inside the <head>
element linking to the below libraries:
-
react.js
file from https://unpkg.com/react@16/umd/react.development.js -
react-dom.js
file from https://unpkg.com/react-dom@16/umd/react-dom.development.js -
browser.js
file from https://cdnjs.cloudflare.com/ajax/libs/babel-core/6.1.19/browser.js
Please get the references to the latest CDN links from here: https://reactjs.org/docs/cdn-links.html. You can use the live CDN links directly or download and keep them in some /js
directory of your project. Note the id="root"
element; it is where the JSX will be rendered.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>React App</title>
<script src="js/react.development.min.js"></script>
<script src="js/react-dom.development.min.js"></script>
<script src="js/browser.min.js"></script>
</head>
<body>
<div id="root"></div>
</body>
</html>
Now ReactJS is made up of parts like components and JSX, about which all you might not have much idea right now. So for your quick conceptualization, below is an example of a simple component called <Hello/>
which returns the JSX <h1>Hello, World!</h1>
:
const Hello = () => {
return (
<h1>Hello, World!</h1>
);
}
This <Hello/>
component along with ReactDOM.render()
function goes inside another <script>
element of type="text/babel"
. The render()
function belongs to the ReactDOM
module which comes from the included library react-dom.js
and takes two arguments:
-
a component (which is
<Hello/>
in our case) -
an HTML element to render the component to (which is
<div id="root">
)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>React App</title>
<script src="js/react.development.min.js"></script>
<script src="js/react-dom.development.min.js"></script>
<script src="js/browser.min.js"></script>
<script type="text/babel">
const Hello = () => {
return (
<h1>Hello, World!</h1>
);
}
ReactDOM.render(<Hello/>, document.getElementById('root'));
</script>
</head>
<body>
<div id="root"></div>
</body>
</html>
The JSX which the component returns gets rendered into the DOM, inside an element with id="root"
.
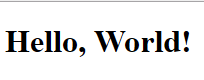