React/ReactJS: Creating Your First React App
In this tutorial, we will learn how to quickly create and launch your very first ReactJS app using the create-react-app
command line tool.
It is assumed that you have Node.js installed in your system, but if not, you can find a detailed list of instructions on how to install it via the package manager here: https://nodejs.org/en/download/package-manager/
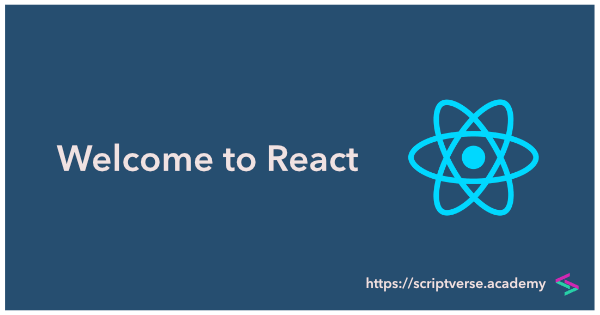
Open you terminal and check the version of Node.js in your system; it should be above 6.
node -v
Install the npx
package runner globally.
npm install -g npx
Get to the directory where you want your app to be and decide a name for it; here I have called it hello-app
, but you can give any other name you please. Type the command
npx create-react-app hello-app
Navigate inside the hello-app
directory just created.
cd hello-app
You will find that there are three directories — node_modules
, public
and src
— and three files — .gitignore
, package.json
and README.md
.
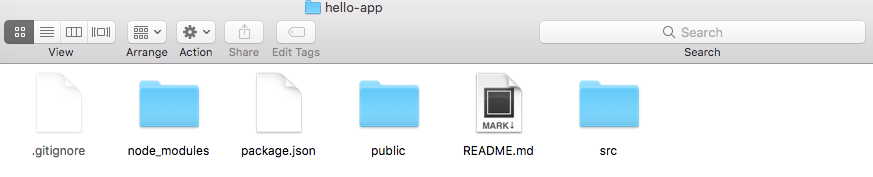
The .gitignore
file lists all the files and directories that should be ignored while committing into the Git repository, including the node_modules
directory which contains all the dependent and sub-dependent packages. The package.json
file contains some project-related metadata and lists all the dependencies and scripts needed for the app. The public
directory contains a favicon (favicon.ico
), a manifest.json
file which provides information about your app and the index.html
file inside which the components would be rendered. The README.md
mark down file contains some 2400+ lines of instructions on configuration, operation, testing and installation and other notes on common bugs and troubleshooting. The src
directory contains the following files by default —
-
App.js
— A default ReactJS component. You may modify/rename/delete it. -
App.css
— Styles for theApp.js
component. You may modify/rename/delete this file also. -
App.test.js
— A test file written for Jest, a Node-based test-runner. -
index.js
— The main file where theApp.js
component is imported and directed to be rendered inside thediv
element withid="root"
inpublic/index.html
via theReactDOM.render()
method. -
index.css
— Styles for theindex.js
file. -
logo.svg
— The light-blue ReactJS logo (you may delete it too later if you do not need it). -
registerServiceWorker.js
— A service worker to cache local assets.
You may create subdirectories and put your JS, CSS, image and test files inside src
.
Run your ReactJS app in the development mode.
npm start
The command automatically launches the app in your default browser. We get our first ReactJS app running.
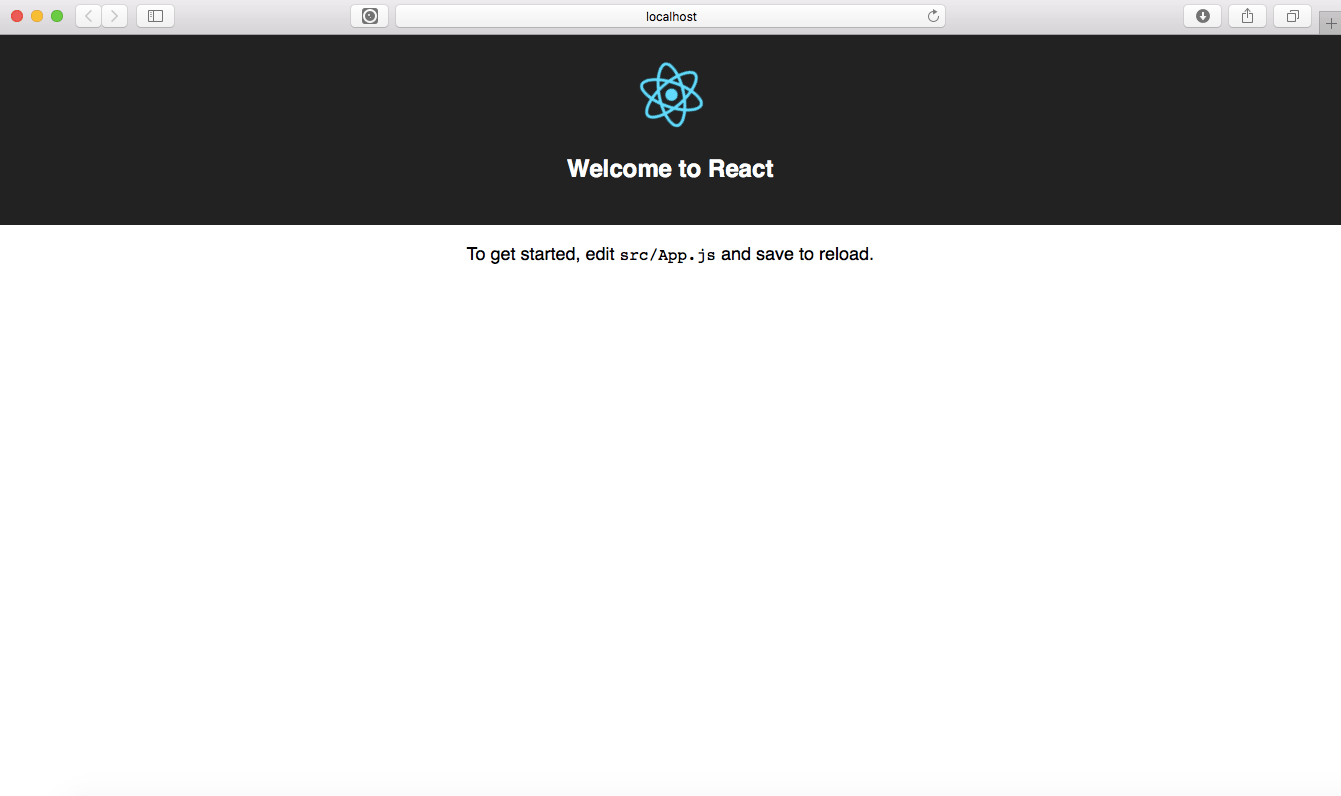
You will notice that it runs on port 3000.

The 'Welcome to React' statement comes from the App.js
file located inside the /src
directory. You can modify its content, rename or even delete it.
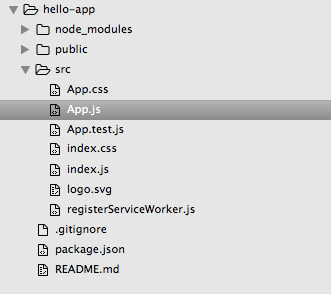
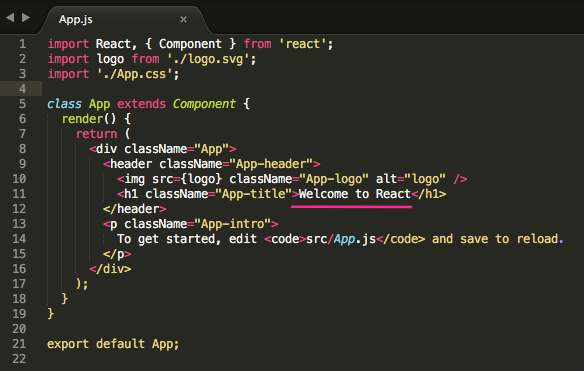
Check your terminal. It flashes the message 'Compiled successfully!' in green and also gives the LAN address along with the port number (http://192.168.0.43:3000
) so that you will be able to access your app even from a phone or tablet connected to the same network.
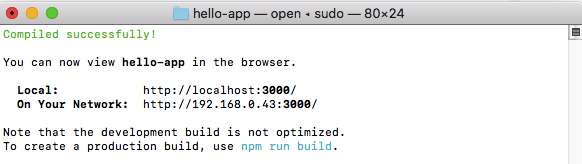
Notes
-
You should NOT rename the following two files:
-
index.html
file inside the/public
directory -
index.js
file inside the/src
directory
-