PHP: 'Hello, World!' Program
As in any programming language, we start by writing our first 'Hello, World!' program in PHP. PHP scripts are mostly run and displayed in a browser, but they can also be run from the command-line.
PHP 'Hello, World!' from the Command Line
Open a text editor (say Gedit, Leafpad, Lime Text or whatever available), create a new file, and type in the below PHP code (or copy & paste it from here)
<?php
echo 'Hello, World!';
?>
Save the file as hello.php
. Below we note some of its basic syntax:
-
PHP code begins with the open tag
<?php
and ends at?>
. -
echo
is a PHP language construct for sending texts/strings, variable values and HTML snippets into the browser. -
Statements in PHP end with a semicolon (
;
). Theecho
statement in the above program ends with a semicolon. -
PHP files have the extension
.php
We can execute the above PHP file from the command line, typing
$ php hello.php
We can also execute PHP as a shell script. Add the #!/usr/bin/php
shebang interpreter directive to the file as its first line of script. The path could be different for different operating systems; type
which php
to confirm. In Mac and Linux systems, it is usually
/usr/bin/php
So, the shell script is
#!/usr/bin/php
<?php
echo 'Hello, World!';
?>
Next, we type a command in the terminal to mark hello.php
as executable
chmod u+x hello.php
The script file can now be executed from the terminal by running the command
./hello.php
PHP 'Hello, World!' in a Browser
In this section, we will embed our PHP 'Hello, World!' script from the above section in HTML, run and see the output in a web browser. The steps are outlined below.
1) Create a PHP project directory
If you are using a Mac, you can go to the working directory created as shown in the Step 3 of our previous tutorial. If you name your working directory as /Sites
, navigate to it
$ cd /users/username/Sites
If you are using a Linux system, navigate to /var/www/html
cd /var/www/html
Now create a project directory there, say, hello
sudo mkdir hello
Navigate again to the newly created hello
directory
cd hello
2) Create hello.php
Open a text editor, create a new file, and type in the below code (or copy & paste it from here) containing our embedded 'Hello, World!' PHP script in the HTML
<!DOCTYPE html>
<html>
<head>
<title>Hello, World! Page</title>
</head>
<body>
<?php
echo 'Hello, World!';
?>
</body>
</html>
Save the file as hello.php
.
3) Start Apache
If you are using a Mac, start the Apache server by typing the below command into the terminal
apachectl start
If you are using a Linux system, type
sudo /etc/init.d/apache2 start
After starting the server, type http://localhost/hello/hello.php
in the URL bar of your web browser. The resulting screen should look something like the one shown below
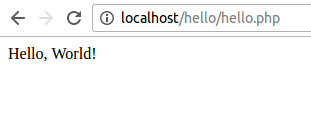
4) View Source Code
Now right-click on the page and select View page source
from the context menu (or just do Ctrl+U)
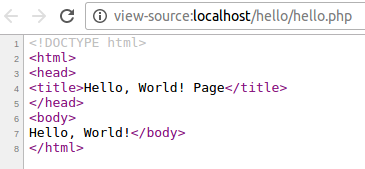
In the page source, we find neither the open tag <?php
nor the end tag ?>
we typed. We do not find the echo
statement either. What happened here?
When the browser sends an HTTP request to the web server for a .php
file, the server first sends the .php
file to the PHP interpreter. The PHP interpreter checks for PHP sections inside the file. When it finds an open tag <?php
, it runs the code inside it till the end tag ?>
, and replaces the code with its output. The server then sends the result back to the browser, which is what we saw on doing Ctrl+U.
Notes
-
A heap of detailed information about your current installed PHP like version, file locations, configuration, predefined variables, environment, HTTP headers, etc can be obtained from the
phpinfo()
function. Just invoke it.<?php phpinfo(); ?>