PHP Form (w/ GET and POST Methods)
In this tutorial, you will learn how to handle HTML forms in PHP and send their data with both GET
and POST
methods to a target page, and access them there with the built-in superglobals $_GET
, $_POST
and $_REQUEST
.
The opening <form>
tag must include the action
and method
attributes.
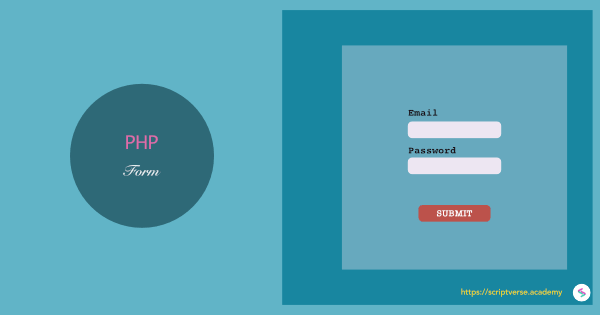
The action
attribute gives the URL or path to the target script, which includes the data from the various form elements inside it. The method
attribute (GET
or POST
) tells the form how the data should be transfered. If the method is set as GET
, then the passed data will be exposed in the URL/address bar of the browser. But the POST
method is secure, and unlike in GET
, data do not get exposed on the URL.
Newsletter Form with GET Method
We first illustrate the GET
method with a simple form snippet for a newsletter. It can be placed inside some element like <footer/>
or a pop-up. We set the method
attribute of the <form/>
tag to GET
and the action
attribute specifies the page to which the filled-in form should be posted. Here, the target page is newsletter.php
. The form contains one <input/>
element of type email
.
<form action="newsletter.php" method="get">
<label>
Email: <input type="email" name="email"/>
</label>
<br/>
<input type="submit" value="Submit"/>
</form>
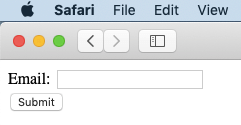
The data passed is the value of the <input/>
element which is filled by the user and which is identified by its name
attribute.
Now the newsletter.php
file could be something like this.
<div>
Thank you. You have been added to our priority mailing list. We will
be sending you information about our latest products, offers and
discounts at <?php echo $_GET['email'];?>
</div>
You might have noticed the $_GET
superblobal in the above script; we learned about in one of our earlier tutorials. This is where it comes in handy. On press of the Submit button, the $_GET
built-in associative array will have fields of the posted form, and will contain an element with key email
, which is the name
attribute of the <input/>
element in the form. On echo
of $_GET['email']
, the posted value is displayed.
We can also use the $_REQUEST
superglobal in place of $_GET
, since it contains the contents of $_GET
, $_POST
and $_COOKIE
.
<div>
Thank you. You have been added to our priority mailing list. We will
be sending you information about our latest products, offers and
discounts at <?php echo $_REQUEST['email'];?>
</div>
Of course, we can also insert validations. If you want the email field to be set first, you can use the isset()
function.
<div>
Thank you. You have been added to our priority mailing list. We will
be sending you information about our latest products, offers and
discounts at <?php if(isset($_GET['email'])) echo $_GET['email'];?>
</div>
Login Form with POST Method
Now for the POST
method, we create a simple login/sign-in form with two <input/>
elements for email and password. The first is of type email
and the second is of type password
. The action
attribute specifies the target page to be account.php
.
<form action="account.php" method="post">
<label>
Email: <input type="email" name="email"/>
</label>
<br/>
<label>
Password: <input type="password" name="password"/>
</label>
<br/>
<input type="submit" value="Submit"/>
</form>
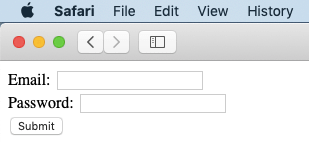
The data passed are the values (filled by the user) which are paired to the name
attributes of the two <input/>
elements.
Now the account.php
target page could be some profile page of yours, where there could be some section where you can view and edit your personal details and such. We will not delve into such complexities of the page. Practically, before accessing your account page, your entered email and password will be validated, probably by connecting to some database and fetching data from it. And for validation, we need to get the passed data in the page. Remember, this is the POST method and no sent data will be exposed on the URL.
The sent data via the POST method can be accessed using the $_POST
superglobal in the account.php
page. We assign them to the variables $email
and $password
, which all can be used in the code later for purposes of fetching data from the database or validation or whatever.
<?php
$email = $_POST['email'];
$password = $_POST['password'];
?>
And as with the $_GET
superglobal above, we can also use the $_REQUEST
method instead of $_POST
.
<?php
$email = $_REQUEST['email'];
$password = $_REQUEST['password'];
?>
Post to the Current Page
Now there will be situations when you would need to post some data to the current page. One-Time Password (OTP) validation is one example. You would not want to move to the next page till the input OTP is validated.
To submit the form on the same page, set the action
attribute of the <form/>
element to <?php echo $_SERVER['PHP_SELF'];?>
.
<form action="<?php echo $_SERVER['PHP_SELF'];?>" method="post">
<label>
OTP: <input type="text" name="otp"/>
</label>
<br/>
<input type="submit" value="Submit"/>
</form>
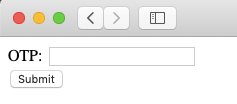
The other way is to leave the action
attribute empty (action=""
).
Notes
-
Use the
POST
method to send sensitive data like passwords. -
If you require the entire query string, you can use
$_SERVER[‘QUERY_STRING’]