Nested Functions
Since a function returns a value, it can also return a function as value. So we can define a function inside another function and return it.
In the example below, we define a function called inner()
, which returns a random number, inside a function called outer()
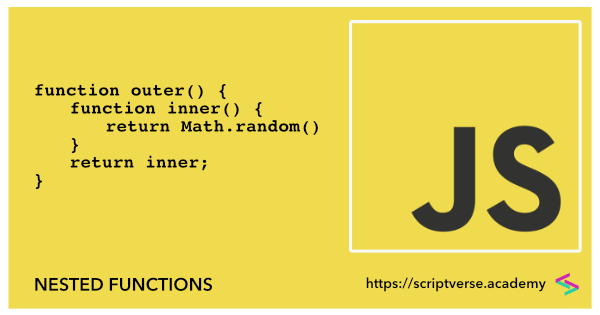
function outer(){
function inner(){
return Math.random();
}
return inner;
}
The same can be written in function literal notation as
var outer = function() {
var inner = function(){
return Math.random();
}
return inner;
}
The inner()
function cannot be accessed directly outside of the outer()
function. But the outer()
function returns a value which can be assigned to a variable, which can then be called as a normal function as shown below
var inside = outer();
inside();
The inner()
function can also be called by using another set of parentheses while calling outer()
as
outer()();
Below, we illustrate the same by passing numerical arguments to both outer()
and inner()
functions and then returning their sum
function outer(x){
function inner(y){
return x+y;
}
return inner;
}
inside(1)
returns the value 2
var inside = outer(1);
inside(1);
which can also be achieved by the following call
outer(1)(1);