window.postMessage()
The window.postMessage()
method gives a provision for sending cross-domain data messages between two browser windows (or a current window and an inner iframe) safely, which otherwise is restricted to the same domain, same protocol, and same port number.
The syntax is as below:
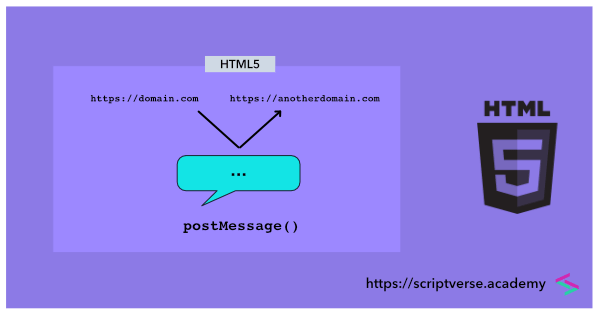
receiverWindow.postMessage(message, domain, transfer);
The receiverWindow
is a reference to the receiver window to which messages will be sent. For example, if there is a single iframe element in a page, it can be accessed as document.getElementsByTagName("iframe")[0].contentWindow
. It can also be a new browser window opened by window.open()
.
The postMessage()
method takes in three arguments as follows
message
domain
transfer
Sender
Using the postMessage()
method, we will be sending a message from here to the other page https://scriptverse.academy/sample/post-message-listener.html, which will be opened via the window.open()
method. The script is as below
//popup window
var domain = 'https://scriptverse.academy';
var popUp = window.open(domain + '/sample/post-message-listener.html','');
//message sender
var message = "Help me, Obi-Wan Kenobi. You're my only hope. - Princess Leia";
popUp.postMessage(message,domain); //sending the message

But instead of a separate page, if you are to post a message to an iframe within, its contentWindow
property is used to reference it, as show here
//popup window
var domain = 'https://scriptverse.academy';
var iframe = document.getElementById('iframeId').contentWindow;
//message sender
var message = "Help me, Obi-Wan Kenobi. You're my only hope. - Princess Leia";
iframe.postMessage(message,domain); //sending the message
Listener/Receiver
The receiver window should set up a listener/handler to the message
event. The e
argument (event) passed to the listener function has the data
property which contains the string/object dispatched by postMessage()
. We check the origin of the message
event, as the event listener could accept messages from any other domain.
window.addEventListener('message', function(e) {
var origin = e.originalEvent.origin || e.origin;
if(origin !== 'https://sciptverse.academy')
return;
console.log('received message: ' + e.data, e);
}, false);
Demo
A little modification is done here on the codes shown above. On the receiver window, instead of printing the message to the console, we print it on the document itself. The OPEN WINDOW
button opens a new browser window on click of it. The SEND MESSAGE
button sends the message across to the targeted window.
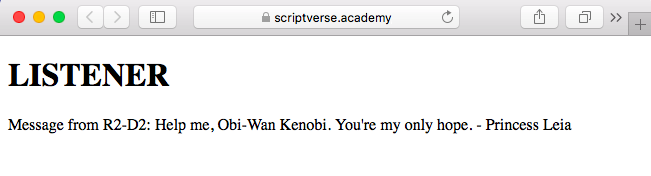